Overview
How does it work?โ
Codehooks is a "no-setup", quick and simple to use backend service, bundling essential features like serverless JavaScript/TypeScript code, a NoSQL database, a Key-Value store, scheduled jobs, queue workers and more.
Everything in Codehooks is organized through Teams (or personal accounts) which again manages projects containing one or more environments (we call them 'spaces').
Each space contains its own separate code and data storage. To manage teams, projects and data, you can use the web-based UI or the CLI - which you install with: npm install -g codehooks
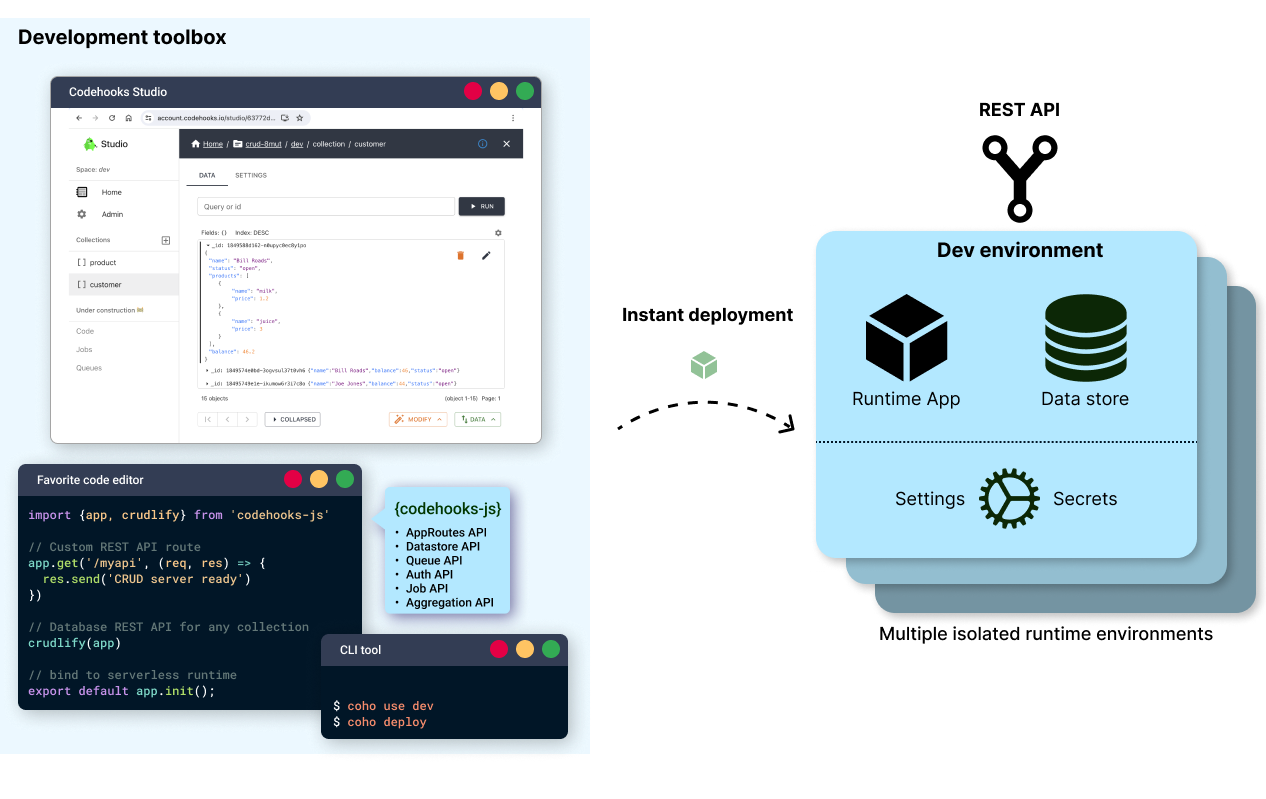
Codehooks Studioโ
- API Dashboard
- Data management
- Security settings
- JSON-schema support
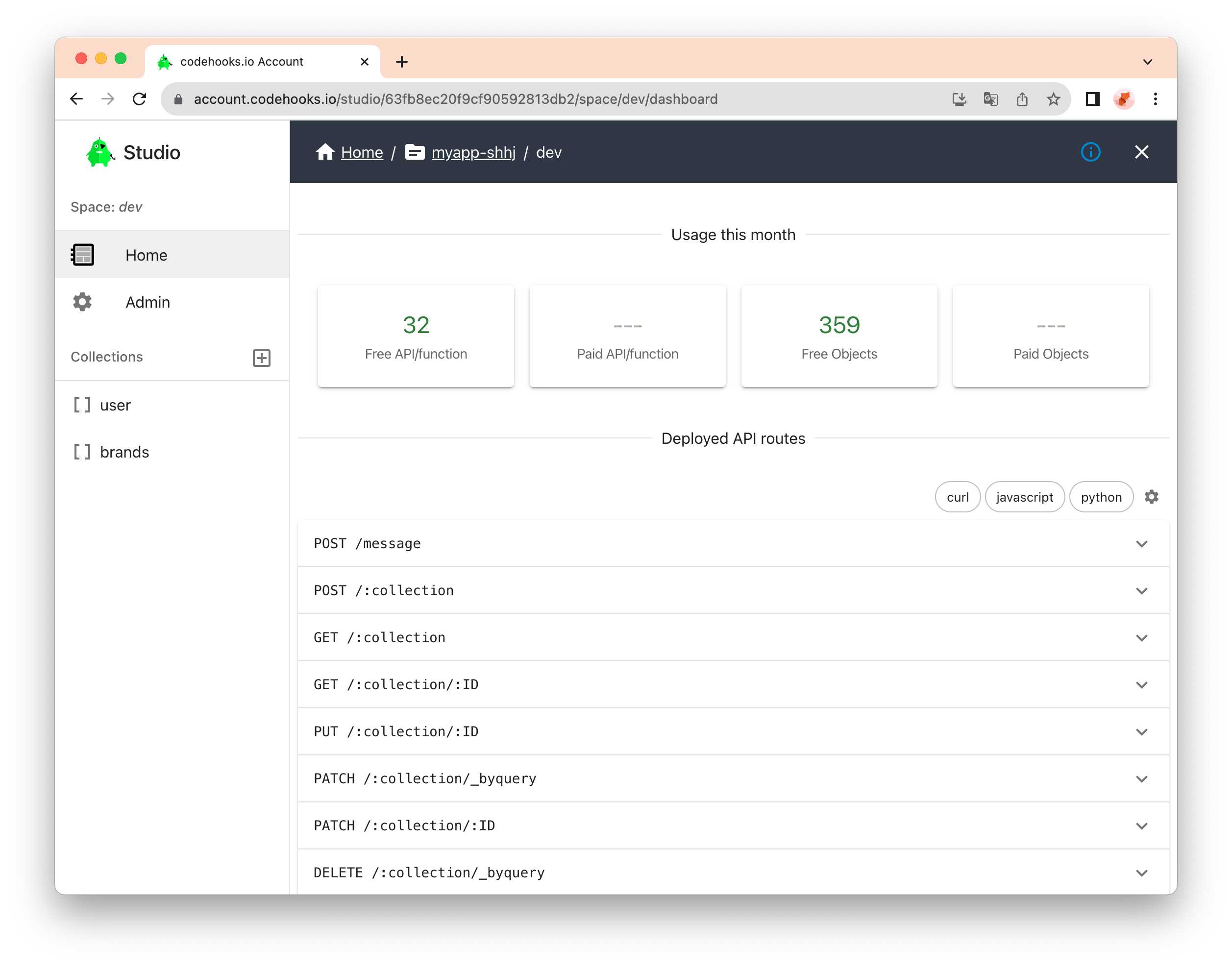
Create data collections and manipulate data with mongoDB query language or the interactive editor. Easy import and export of CSV or JSON data.
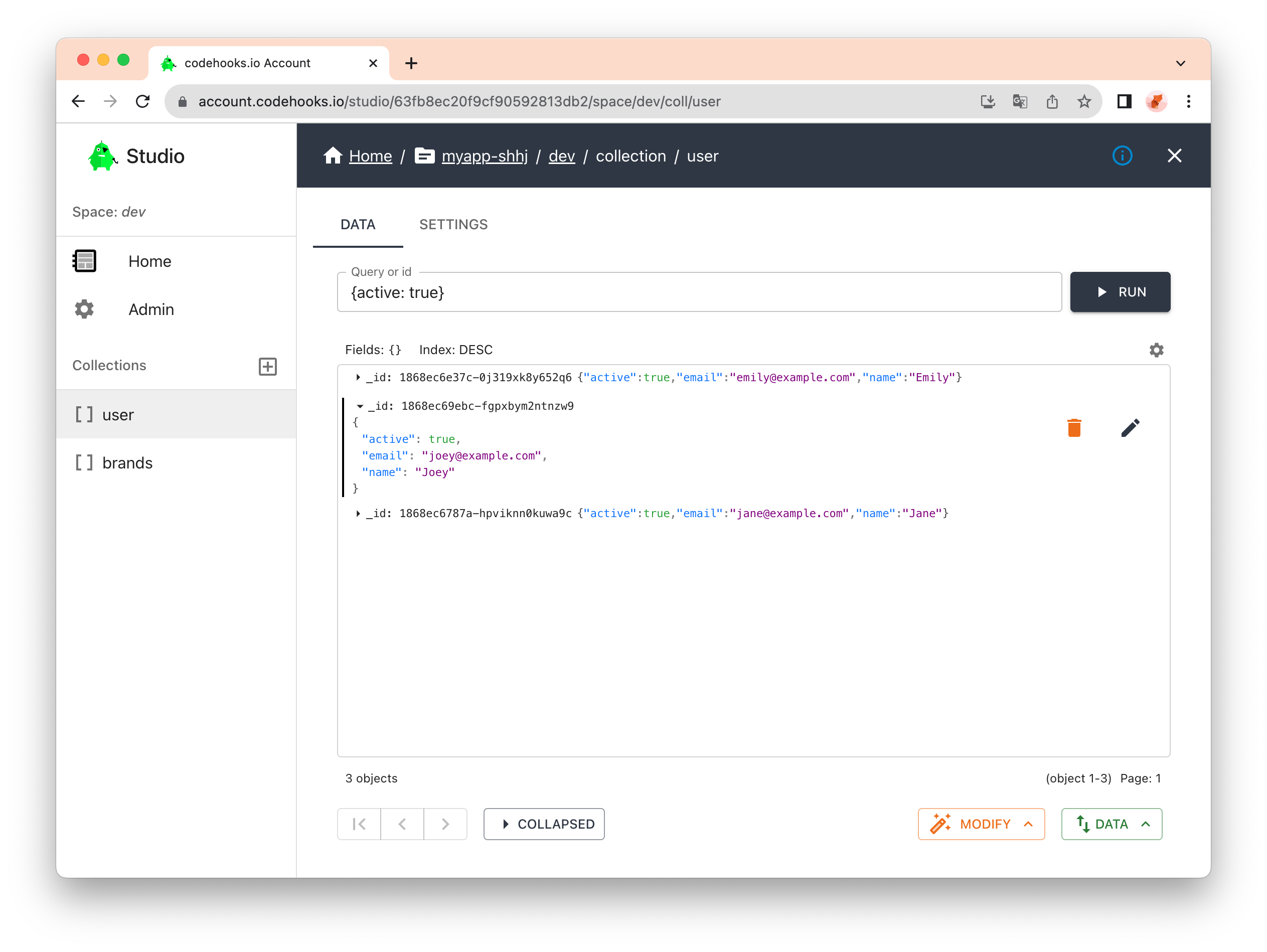
Protect access to your database with API tokens, JWT tokens and IP filters. Create runtime application environment variables and security settings.
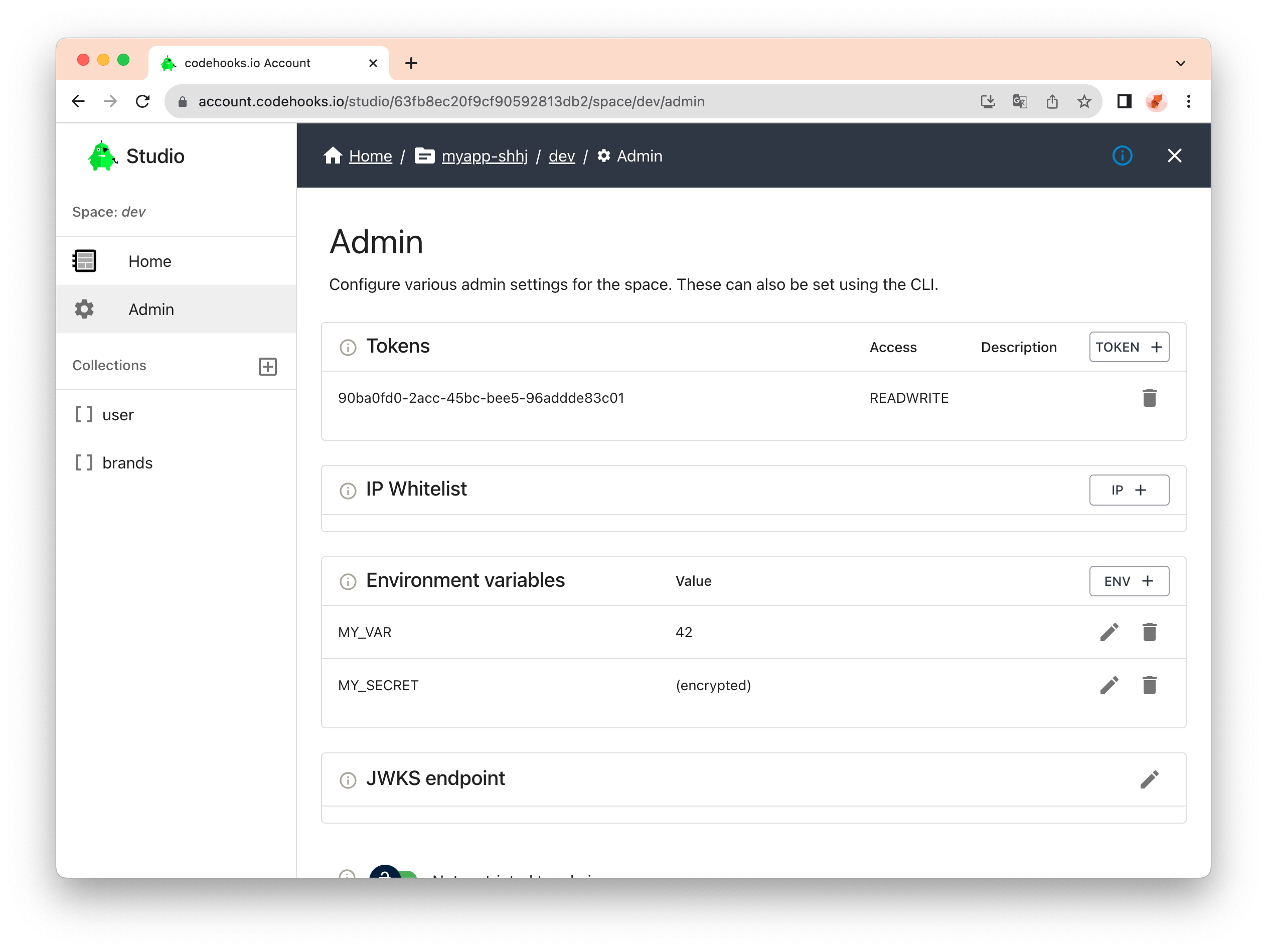
Keep your data consistent and validated using JSON-schema directly on the database level.
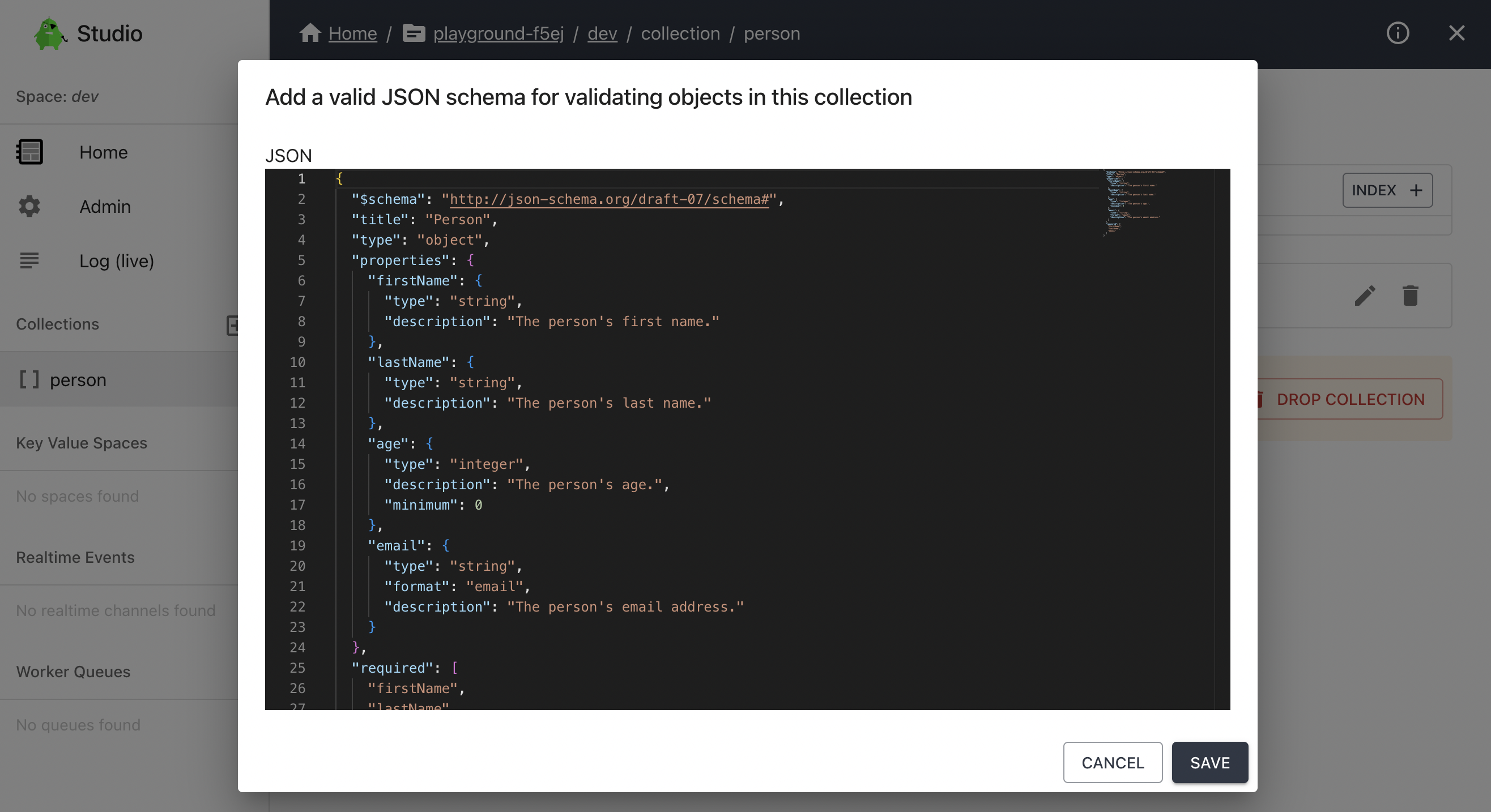
codehooks-jsโ
The codehooks-js
library is the foundation for all Codehooks JavaScript development.
This library contains a set of 6 specific APIs which simplifies and accelerates backend development.
REST, CRUD or custom APIs
NoSQL & Key-Value
Code triggered by CRON
Long running processes
Serve static content
Groups & sums
API developmentโ
Get a flying start with an automatic CRUD REST API
myproject-fafb
. Under the hood, Codehooks deploys an automatic REST API which is made up of the following really short - but complete application code:import {app} from 'codehooks-js'
// Use Crudlify to create a REST API for any database collection
app.crudlify()
// bind to serverless runtime
export default app.init();
const URL = 'https://myproject-fafb.api.codehooks.io/dev/orders';
response = await fetch(URL+'?status=OPEN', /* options */)
const result = await response.json()
NoSQL and Key-Value Datastoresโ
Easy database API inspired by MongoDB
import {app, datastore} from 'codehooks-js'
// REST API route
app.get('/sales', async (req, res) => {
const conn = await datastore.open();
const query = { status: "OPEN" };
const options = { sort: { date: 1 } }
conn.getMany('orders', query, options).json(res); // stream JSON to client
})
// bind to serverless runtime
export default app.init();
Speed up your application with a Key-Value cache
ttl
option.import {app, datastore} from 'codehooks-js'
// REST API route
app.post('/cacheit', async (req, res) => {
const conn = await Datastore.open();
const opt = {ttl: 60*1000};
await conn.set('my_cache_key', '1 min value', opt);
res.send('Look dad, I have my own cache now!');
})
// bind to serverless runtime
export default app.init();
Background Jobsโ
CRON expressions + your function keeps running
In the simple example below, the CRON expression */5 * * * *
will trigger a JavaScript function each 5 minutes.
import app from 'codehooks-js'
// Trigger a function with a CRON expression
app.job('*/5 * * * *', (_, job) => {
console.log('Hello every 5 minutes')
// Do stuff ...
job.end()
});
export default app.init();
Queuesโ
Scale workloads with queues and worker functions
Check out the example below, where a REST API request starts a complex task by adding it to the Queue for later processing.
import { app, Datastore } from 'codehooks-js'
// register a Queue task worker
app.worker('ticketToPDF', (req, res) => {
const { email, ticketid } = req.body.payload;
//TODO: implement code to fetch ticket data, produce PDF
// and send email with attachment using Mailgun, Sendgrid or Amazon SES
// ...and implement error handling
res.end(); // done processing queue item
})
// REST API
app.post('/createticket', async (req, res) => {
const { email, ticketid } = req.body;
const conn = await Datastore.open();
await conn.enqueue("ticketToPDF", { email, ticketid });
res.status(201).json({"message": `Check email ${email}`});
})
export default app.init();
File System & Blob storageโ
Serve any document or file content
dev
space (environment) of the project. You can publish documents and files in two ways. Use the file-upload CLI command for mass uploads of files/directories:$ coho file-upload --projectname 'myproject-fafc' --space 'dev' --src '/somedir/' --target '/myblobs'
$ coho deploy
import {app} from 'codehooks-js'
// serve the assets directory of the deployed project source code
app.static({directory: "/assets"})
// serve a direcory of Blob storage files
app.storage({route:"/documents", directory: "/myblobs"})
// CRUD REST API
crudlify(app)
// bind to serverless runtime
export default app.init();
Data Aggregationโ
Easy to use data aggregation format
import { app, Datastore, aggregation } from 'codehooks-js'
... // collapsed code
const spec = {
$group: {
$field: "month",
$max: ["sales", "winback"],
$sum: "sales"
}
}
const db = await datastore.open();
const dbstream = db.getMany('salesData');
const result = await aggregation(dbstream, spec)
... // collapsed code
{
"oct": {
"sales": {
"max": 1477.39,
"sum": 1234.05
},
"winback": {
"max": 22.0
}
},
"nov": {
"sales": {
"max": 2357.00,
"sum": 5432.00
},
"winback": {
"max": 91.0
}
},
}
Next Stepsโ
Read this far? Great ๐
It's time to get your feet wet ๐ฆ. Why don't you start thinking about how codehooks' toolbox can help you build your solution. You can get started with the quickstart for developers or just sign up and create your project and space using the web UI. You can easily upload some CSV data and immediately test your new API.
The documentation or tutorials are great places to start. If you need more help, feel free to contact us on the chat.